Getting Started with GraphQL - A Revolutionary API Language

GraphQL is a new way of creating APIs that changes the way we work with them. In this article, I will introduce you to what GraphQL is and how it compares to traditional REST APIs. We’ll also look at how to use GraphQL with NodeJS.
What is GraphQL? In simple terms, GraphQL is a query language for APIs. It allows the client to specify what data it wants to receive from the server, rather than being limited to pre-defined endpoints or receiving excess data.
GraphQL can also combine multiple data types in one request, eliminating the need for multiple REST APIs. Developed by Facebook internally in 2012 and released publicly in 2015, you can learn more about GraphQL on their official website. GraphQL can be used with many programming languages, but we’ll focus on NodeJS for this article.
First, I will create a NodeJS Express project using Typescript. You can refer to this article for detailed steps on how to set up the project, or follow these commands in order:
npm init -y
npm install nodemon express graphql express-graphql
Create a server.js
file in the project's root directory and configure it like so:
import express from 'express';
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Demo NodeJS GraphQL application!');
});
app.listen(port, () => {
console.log(`Server is listening on port ${port}`);
});
Configure the application in package.json
with this script:
"scripts": {
"start": "nodemon server.js"
}
Start the application with npm start
and verify it works by visiting http://localhost:3000 in your web browser.
GraphQL Schema
In GraphQL, there is only one endpoint for all requests: /graphql
. However, requests will have separate requirements, so we define a schema to handle multiple tasks.
The schema serves several purposes:
- Define specific endpoints
- Specify input and output fields for each request
- Determine which actions should be called with each request
// query.ts
import {GraphQLObjectType, GraphQLString} from 'graphql';
export const queryType = new GraphQLObjectType({
name: 'Query',
fields: {
hello: {
type: GraphQLString,
resolve: () => {
return "Hello world!";
}
}
}
});
In the above example, fields
defines an endpoint (/hello
) with a corresponding data type (GraphQLString
). The resolve()
function handles requests to this endpoint. In this case, it simply returns the string "Hello world!".
GraphQL Installation and Configuration
We use graphqlHTTP
from the express-graphql
library to create a GraphQL server at this endpoint:
// server.ts
import { graphqlHTTP } from 'express-graphql';
import { GraphQLSchema } from 'graphql';
import { queryType } from './query';
const schema = new GraphQLSchema({ query: queryType });
app.use('/graphql', graphqlHTTP({
schema: schema,
graphiql: true,
}));
Now visit http://localhost:3000/graphql in your web browser to test GraphQL queries. For example, entering the following query in the left query frame will result in the response below:

Adding GraphQL Endpoints
To let us experience and appreciate more of the advantages of GraphQL, I will create another example that is a bit more complex.
Here I will define an endpoint that takes a request param called id
and returns a movie
object (with fields id
, name
, and year
).
Note: this is just a simple example to show you how to use GraphQL to define an endpoint that takes a request param and returns an object. You can use this example as a reference to define more complex endpoints according to your needs.
import {GraphQLID, GraphQLInt, GraphQLObjectType, GraphQLString} from 'graphql';
export const queryType = new GraphQLObjectType({
name: 'Query',
fields: {
movie: {
type: new GraphQLObjectType({
name: 'movieType',
fields: {
id: {type: GraphQLID},
name: {type: GraphQLString},
year: {type: GraphQLInt},
},
}),
args: {
id: {type: GraphQLInt},
},
resolve: (source, args) => {
return {id: args.id, name: 'name', year: 1987}
},
},
},
})
Again, pass queryType
to the schema:
const schema = new GraphQLSchema({ query: queryType });
and run a query like so:
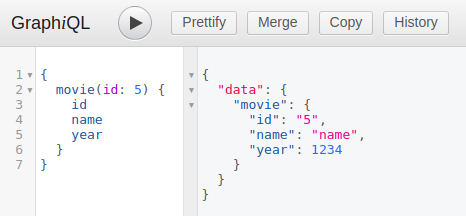
Tip
You can define both endpoints at the same time like this:
import {
GraphQLID,
GraphQLInt,
GraphQLObjectType,
GraphQLString,
} from "graphql";
export const queryType = new GraphQLObjectType({
name: "Query",
fields: {
hello: {
type: GraphQLString,
resolve: () => "Hello World!",
},
movie: {
type: new GraphQLObjectType({
name: "movieType",
fields: {
id: { type: GraphQLID },
name: { type: GraphQLString },
year: { type: GraphQLInt },
},
}),
args: {
id: { type: GraphQLInt },
},
resolve: (source, args) => {
return { id: args.id, name: "name", year: 1987 };
},
},
},
});
In this article, we explained what GraphQL is and how it compares to REST APIs. We also demonstrated how to use GraphQL with NodeJS by building a simple project. With its speed and flexibility, GraphQL is likely to become an important tool for developers creating APIs. Its ability to provide only the data that the client needs and allow for multiple data sources in one request makes it a powerful alternative to traditional REST APIs.
In conclusion, if you are looking for a new way to build APIs or just want to stay up-to-date on the latest developments, learning GraphQL is definitely worth your time. With its revolutionary approach and growing popularity, GraphQL is shaping up to be an important technology for years to come.